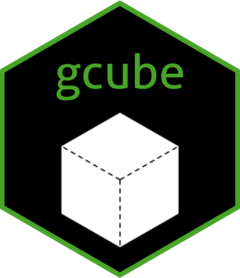
Map a cube simulation function over multiple rows of a dataframe
Source:R/map_simulation_functions.R
map_simulation_functions.Rd
This function executes a cube simulation function (simulate_occurrences()
,
sample_observations()
, filter_observations()
,
add_coordinate_uncertainty()
, or grid_designation()
) over multiple rows
of a dataframe with potentially different function arguments over multiple
columns.
Arguments
- f
One of five cube simulation functions:
simulate_occurrences()
,sample_observations()
,filter_observations()
,add_coordinate_uncertainty()
, orgrid_designation()
.- df
A dataframe containing multiple rows, each representing a different species. The columns are function arguments with values used for mapping
f
for each species. Columns not used by this function will be retained in the output.- nested
Logical. If
TRUE
(default), retains list-column containing dataframes calculated byf
. Otherwise, expands this list-column into rows and columns.- progress
Logical. Whether to show a progress bar. Set to
TRUE
to display a progress bar,FALSE
(default) to suppress it.
Value
In case of nested = TRUE
, a dataframe identical to df
, with an
extra list-column called mapped_col
containing an sf object for each row
computed by the function specified in f
. In case of nested = FALSE
, this
list-column is expanded into additional rows and columns.
Examples
# Load packages
library(sf)
library(dplyr)
# Create polygon
plgn <- st_polygon(list(cbind(c(5, 10, 8, 2, 3, 5), c(2, 1, 7, 9, 5, 2))))
## Example with simple column names
# Specify dataframe for 3 species with custom function arguments
species_dataset_df <- tibble(
taxonID = c("species1", "species2", "species3"),
species_range = rep(list(plgn), 3),
initial_average_occurrences = c(50, 100, 500),
n_time_points = rep(6, 3),
temporal_function = c(simulate_random_walk, simulate_random_walk, NA),
sd_step = c(1, 1, NA),
spatial_pattern = "random",
seed = 123)
# Simulate occurrences
sim_occ_raw <- map_simulation_functions(
f = simulate_occurrences,
df = species_dataset_df)
#> [1] [using unconditional Gaussian simulation]
#> [2] [using unconditional Gaussian simulation]
#> [3] [using unconditional Gaussian simulation]
sim_occ_raw
#> # A tibble: 3 × 9
#> taxonID species_range initial_average_occur…¹ n_time_points temporal_function
#> <chr> <list> <dbl> <dbl> <list>
#> 1 species1 <XY> 50 6 <fn>
#> 2 species2 <XY> 100 6 <fn>
#> 3 species3 <XY> 500 6 <lgl [1]>
#> # ℹ abbreviated name: ¹initial_average_occurrences
#> # ℹ 4 more variables: sd_step <dbl>, spatial_pattern <chr>, seed <dbl>,
#> # mapped_col <list>
# Unnest output and create sf object
sim_occ_raw_unnested <- map_simulation_functions(
f = simulate_occurrences,
df = species_dataset_df,
nested = FALSE)
#> [1] [using unconditional Gaussian simulation]
#> [2] [using unconditional Gaussian simulation]
#> [3] [using unconditional Gaussian simulation]
sim_occ_raw_unnested %>%
st_sf()
#> Simple feature collection with 3936 features and 10 fields
#> Geometry type: POINT
#> Dimension: XY
#> Bounding box: xmin: 2.006092 ymin: 1.000961 xmax: 9.980644 ymax: 8.991332
#> CRS: NA
#> # A tibble: 3,936 × 11
#> taxonID species_range initial_average_occu…¹ n_time_points temporal_function
#> <chr> <list> <dbl> <dbl> <list>
#> 1 species1 <XY> 50 6 <fn>
#> 2 species1 <XY> 50 6 <fn>
#> 3 species1 <XY> 50 6 <fn>
#> 4 species1 <XY> 50 6 <fn>
#> 5 species1 <XY> 50 6 <fn>
#> 6 species1 <XY> 50 6 <fn>
#> 7 species1 <XY> 50 6 <fn>
#> 8 species1 <XY> 50 6 <fn>
#> 9 species1 <XY> 50 6 <fn>
#> 10 species1 <XY> 50 6 <fn>
#> # ℹ 3,926 more rows
#> # ℹ abbreviated name: ¹initial_average_occurrences
#> # ℹ 6 more variables: sd_step <dbl>, spatial_pattern <chr>, seed <dbl>,
#> # time_point <int>, sampling_p1 <dbl>, geometry <POINT>