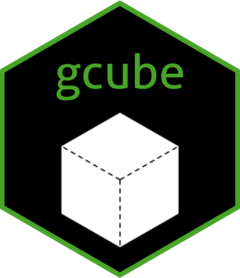
Observations to grid designation to create a data cube
Source:R/grid_designation.R
grid_designation.Rd
This function designates observations to cells of a given grid to create an aggregated data cube.
Arguments
- observations
An sf object with POINT geometry and a
time_point
andcoordinateUncertaintyInMeters
column. If the former column is not present, the function will assume a single time point. If the latter column is not present, the function will assume no uncertainty (zero meters) around the observation points.- grid
An sf object with POLYGON geometry (usually a grid) to which observations should be designated.
- id_col
The column name containing unique IDs for each grid cell. If
"row_names"
(the default), a new columncell_code
is created where the row names represent the unique IDs.- missing_uncertainty
A positive numeric value (default: 1000 m) used to replace missing (
NA
) values in thecoordinateUncertaintyInMeters
column. This ensures that all observations have a defined uncertainty radius for sampling. Only applied when the column is present but containsNA
values; if the column itself is absent, a value of 0 is assumed instead.- seed
A positive numeric value setting the seed for random number generation to ensure reproducibility. If
NA
(default), thenset.seed()
is not called at all. If notNA
, then the random number generator state is reset (to the state before calling this function) upon exiting this function.- aggregate
Logical. If
TRUE
(default), returns data cube in aggregated form (grid with the number of observations per grid cell). Otherwise, returns sampled points within the uncertainty circle.- randomisation
Character. Method used for sampling within the uncertainty circle around each observation.
"uniform"
(default) means each point in the uncertainty circle has an equal probability of being selected. The other option is"normal"
, where a point is sampled from a bivariate Normal distribution with means equal to the observation point and variance such thatp_norm
% of all possible samples from this Normal distribution fall within the uncertainty circle. Seesample_from_binormal_circle()
.- p_norm
A numeric value between 0 and 1, used only if
randomisation = "normal"
. The proportion of all possible samples from a bivariate Normal distribution that fall within the uncertainty circle. Default is 0.95.
Value
If aggregate = TRUE
, an sf object with POLYGON geometry
containing the grid cells, an n
column with the number of observations per
grid cell, and a min_coord_uncertainty
column with the minimum coordinate
uncertainty per grid cell. If aggregate = FALSE
, an sf object with POINT
geometry containing the sampled observations within the uncertainty circles,
and a coordinateUncertaintyInMeters
column with the coordinate uncertainty
for each observation.
See also
Other main:
add_coordinate_uncertainty()
,
filter_observations()
,
sample_observations()
,
simulate_occurrences()
,
virtualsample_to_sf()
Examples
library(sf)
library(dplyr)
# Create four random points
n_points <- 4
xlim <- c(3841000, 3842000)
ylim <- c(3110000, 3112000)
coordinate_uncertainty <- rgamma(n_points, shape = 5, rate = 0.1)
observations_sf <- data.frame(
lat = runif(n_points, ylim[1], ylim[2]),
long = runif(n_points, xlim[1], xlim[2]),
time_point = 1,
coordinateUncertaintyInMeters = coordinate_uncertainty
) %>%
st_as_sf(coords = c("long", "lat"), crs = 3035)
# Add buffer uncertainty in meters around points
observations_buffered <- observations_sf %>%
st_buffer(observations_sf$coordinateUncertaintyInMeters)
# Create grid
grid_df <- st_make_grid(
observations_buffered,
square = TRUE,
cellsize = c(200, 200)
) %>%
st_sf()
# Create occurrence cube
grid_designation(
observations = observations_sf,
grid = grid_df,
seed = 123
)
#> Simple feature collection with 40 features and 4 fields
#> Geometry type: POLYGON
#> Dimension: XY
#> Bounding box: xmin: 3841029 ymin: 3110005 xmax: 3841829 ymax: 3112005
#> Projected CRS: ETRS89-extended / LAEA Europe
#> # A tibble: 40 × 5
#> time_point cell_code n min_coord_uncertainty geometry
#> * <dbl> <chr> <int> <dbl> <POLYGON [m]>
#> 1 1 1 1 59.9 ((3841029 3110005, 3841229 …
#> 2 1 35 1 83.1 ((3841429 3111605, 3841629 …
#> 3 1 37 1 26.1 ((3841029 3111805, 3841229 …
#> 4 1 4 1 38.5 ((3841629 3110005, 3841829 …
#> 5 1 2 0 NA ((3841229 3110005, 3841429 …
#> 6 1 3 0 NA ((3841429 3110005, 3841629 …
#> 7 1 5 0 NA ((3841029 3110205, 3841229 …
#> 8 1 6 0 NA ((3841229 3110205, 3841429 …
#> 9 1 7 0 NA ((3841429 3110205, 3841629 …
#> 10 1 8 0 NA ((3841629 3110205, 3841829 …
#> # ℹ 30 more rows