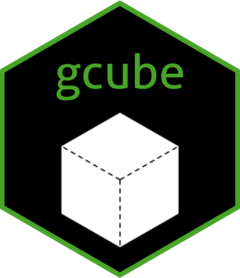
Sample from a circle using the Uniform distribution
Source:R/sample_from_uniform_circle.R
sample_from_uniform_circle.Rd
This function samples a new observations point of a species within the uncertainty circle around each observation assuming a Uniform distribution.
Arguments
- observations
An sf object with POINT geometry and a
time_point
andcoordinateUncertaintyInMeters
column. If the former column is not present, the function will assume a single time point. If the latter column is not present, the function will assume no uncertainty (zero meters) around the observation points.- missing_uncertainty
A positive numeric value (default: 1000 m) used to replace missing (
NA
) values in thecoordinateUncertaintyInMeters
column. This ensures that all observations have a defined uncertainty radius for sampling. Only applied when the column is present but containsNA
values; if the column itself is absent, a value of 0 is assumed instead.- seed
A positive numeric value setting the seed for random number generation to ensure reproducibility. If
NA
(default), thenset.seed()
is not called at all. If notNA
, then the random number generator state is reset (to the state before calling this function) upon exiting this function.
Value
An sf object with POINT geometry containing the locations of the
sampled occurrences and a coordinateUncertaintyInMeters
column containing
the coordinate uncertainty for each observation.
See also
Other designation:
sample_from_binormal_circle()
Examples
library(sf)
library(dplyr)
# Create four random points
n_points <- 4
xlim <- c(3841000, 3842000)
ylim <- c(3110000, 3112000)
coordinate_uncertainty <- rgamma(n_points, shape = 5, rate = 0.1)
observations_sf <- data.frame(
lat = runif(n_points, ylim[1], ylim[2]),
long = runif(n_points, xlim[1], xlim[2]),
time_point = 1,
coordinateUncertaintyInMeters = coordinate_uncertainty
) %>%
st_as_sf(coords = c("long", "lat"), crs = 3035)
# Sample points within uncertainty circles according to uniform rules
sample_from_uniform_circle(
observations = observations_sf,
seed = 123
)
#> Simple feature collection with 4 features and 2 fields
#> Geometry type: POINT
#> Dimension: XY
#> Bounding box: xmin: 3841383 ymin: 3110074 xmax: 3841859 ymax: 3111938
#> Projected CRS: ETRS89-extended / LAEA Europe
#> # A tibble: 4 × 3
#> # Rowwise:
#> time_point coordinateUncertaintyInMeters geometry
#> <dbl> <dbl> <POINT [m]>
#> 1 1 25.0 (3841383 3110460)
#> 2 1 111. (3841446 3111938)
#> 3 1 7.30 (3841606 3110807)
#> 4 1 37.7 (3841859 3110074)