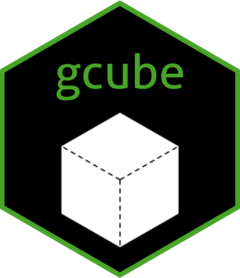
Map filter_observations()
over multiple species
Source: R/map_filter_observations.R
map_filter_observations.Rd
This function executes filter_observations()
over multiple rows of a
dataframe, representing different species, with potentially
different function arguments over multiple columns.
Arguments
- df
A dataframe containing multiple rows, each representing a different species. The columns are function arguments with values used for mapping
filter_observations()
for each species. Columns not used by this function will be retained in the output.- nested
Logical. If
TRUE
(default), retains list-column containing sf objects/dataframes calculated byfilter_observations()
. Otherwise, expands this list-column into rows and columns.- arg_list
A named list or
NA
. IfNA
(default), the function assumes column names indf
are identical to argument names offilter_observations()
. If column names differ, they must be specified as a named list where the names are the argument names offilter_observations()
, and the associated values are the corresponding column names indf
.
Value
In case of nested = TRUE
, a dataframe identical to df
, with an
extra list-column called observations
containing an sf object with POINT
geometry or simple dataframe for each row computed by
filter_observations()
. In case of nested = FALSE
, this list-column is
expanded into additional rows and columns.
See also
Other multispecies:
generate_taxonomy()
,
map_add_coordinate_uncertainty()
,
map_grid_designation()
,
map_sample_observations()
,
map_simulate_occurrences()
Examples
# Load packages
library(sf)
library(dplyr)
# Create polygon
plgn <- st_polygon(list(cbind(c(5, 10, 8, 2, 3, 5), c(2, 1, 7, 9, 5, 2))))
## Example with simple column names
# Specify dataframe for 3 species with custom function arguments
species_dataset_df <- tibble(
taxonID = c("species1", "species2", "species3"),
species_range = rep(list(plgn), 3),
initial_average_occurrences = c(50, 100, 200),
n_time_points = rep(6, 3),
temporal_function = c(simulate_random_walk, simulate_random_walk, NA),
sd_step = c(1, 1, NA),
spatial_pattern = "random",
detection_probability = c(0.8, 0.9, 1),
invert = FALSE,
seed = 123)
# Simulate occurrences
sim_occ1 <- map_simulate_occurrences(df = species_dataset_df)
#> [1] [using unconditional Gaussian simulation]
#> [2] [using unconditional Gaussian simulation]
#> [3] [using unconditional Gaussian simulation]
# Sample observations
samp_obs1 <- map_sample_observations(df = sim_occ1)
# Filter observations
filter_obs_nested <- map_filter_observations(df = samp_obs1)
filter_obs_nested
#> # A tibble: 3 × 13
#> taxonID species_range initial_average_occur…¹ n_time_points temporal_function
#> <chr> <list> <dbl> <dbl> <list>
#> 1 species1 <XY> 50 6 <fn>
#> 2 species2 <XY> 100 6 <fn>
#> 3 species3 <XY> 200 6 <lgl [1]>
#> # ℹ abbreviated name: ¹initial_average_occurrences
#> # ℹ 8 more variables: sd_step <dbl>, spatial_pattern <chr>,
#> # detection_probability <dbl>, invert <lgl>, seed <dbl>, occurrences <list>,
#> # observations_total <list>, observations <list>
## Example with deviating column names
# Specify dataframe for 3 species with custom function arguments
species_dataset_df2 <- species_dataset_df %>%
rename(polygon = species_range,
sd = sd_step,
det_prob = detection_probability,
inv = invert)
# Create named list for argument conversion
arg_conv_list <- list(
species_range = "polygon",
sd_step = "sd",
detection_probability = "det_prob",
invert = "inv"
)
# Simulate occurrences
sim_occ2 <- map_simulate_occurrences(
df = species_dataset_df2,
arg_list = arg_conv_list)
#> [1] [using unconditional Gaussian simulation]
#> [2] [using unconditional Gaussian simulation]
#> [3] [using unconditional Gaussian simulation]
# Sample observations
samp_obs2 <- map_sample_observations(
df = sim_occ2,
arg_list = arg_conv_list)
# Filter observations
map_filter_observations(
df = samp_obs2,
arg_list = arg_conv_list)
#> # A tibble: 3 × 13
#> taxonID polygon initial_average_occur…¹ n_time_points temporal_function sd
#> <chr> <list> <dbl> <dbl> <list> <dbl>
#> 1 species1 <XY> 50 6 <fn> 1
#> 2 species2 <XY> 100 6 <fn> 1
#> 3 species3 <XY> 200 6 <lgl [1]> NA
#> # ℹ abbreviated name: ¹initial_average_occurrences
#> # ℹ 7 more variables: spatial_pattern <chr>, det_prob <dbl>, inv <lgl>,
#> # seed <dbl>, occurrences <list>, observations_total <list>,
#> # observations <list>