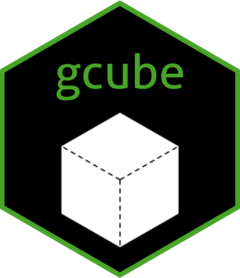
Apply manual sampling bias to occurrences via a grid
Source:R/apply_manual_sampling_bias.R
apply_manual_sampling_bias.Rd
This function adds a sampling bias weight column to an sf object containing occurrences. The sampling probabilities are based on bias weights within each cell of a provided grid layer.
Arguments
- occurrences_sf
An sf object with POINT geometry representing the occurrences.
- bias_weights
An
sf
object with POLYGON geometry representing the grid with bias weights. This sf object should contain abias_weight
column and ageometry
column. Higher weights indicate a higher probability of sampling. Weights must be numeric values between 0 and 1 or positive integers, which will be rescaled to values between 0 and 1.
Value
An sf object with POINT geometry that includes a bias_weight
column containing the sampling probabilities based on the sampling bias.
See also
Other detection:
apply_polygon_sampling_bias()
Examples
# Load packages
library(sf)
#> Linking to GEOS 3.12.1, GDAL 3.8.4, PROJ 9.4.0; sf_use_s2() is TRUE
library(dplyr)
#>
#> Attaching package: ‘dplyr’
#> The following objects are masked from ‘package:stats’:
#>
#> filter, lag
#> The following objects are masked from ‘package:base’:
#>
#> intersect, setdiff, setequal, union
library(ggplot2)
# Create polygon
plgn <- st_polygon(list(cbind(c(5, 10, 8, 2, 3, 5), c(2, 1, 7, 9, 5, 2))))
# Get occurrence points
occurrences_sf <- simulate_occurrences(plgn)
#> [using unconditional Gaussian simulation]
# Create grid with bias weights
grid <- st_make_grid(
plgn,
n = c(10, 10),
square = TRUE) %>%
st_sf()
grid$bias_weight <- runif(nrow(grid), min = 0, max = 1)
# Calculate occurrence bias
occurrence_bias <- apply_manual_sampling_bias(occurrences_sf, grid)
occurrence_bias
#> Simple feature collection with 40 features and 3 fields
#> Geometry type: POINT
#> Dimension: XY
#> Bounding box: xmin: 2.805565 ymin: 1.46267 xmax: 8.254267 ymax: 8.551619
#> CRS: NA
#> First 10 features:
#> time_point sampling_p1 bias_weight geometry
#> 40 1 0.9214596 0.23581957 POINT (7.10732 1.658796)
#> 5 1 0.9993232 0.13919488 POINT (7.90152 1.46267)
#> 13 1 0.9979411 0.04815941 POINT (6.158066 1.950062)
#> 31 1 0.9950043 0.04815941 POINT (6.102045 2.024401)
#> 25 1 0.9998381 0.68258195 POINT (7.045201 1.830053)
#> 4 1 0.9782428 0.62286399 POINT (7.956353 1.897629)
#> 14 1 0.6684262 0.55225256 POINT (4.923372 3.282674)
#> 27 1 0.9999701 0.55225256 POINT (4.751308 2.751113)
#> 28 1 0.9987659 0.55225256 POINT (4.98449 2.713699)
#> 23 1 0.9926203 0.77067736 POINT (5.477754 3.061427)
# Visualise where the bias is
ggplot() +
geom_sf(data = plgn) +
geom_sf(data = grid, alpha = 0) +
geom_sf(data = occurrence_bias, aes(colour = bias_weight)) +
geom_sf_text(data = grid, aes(label = round(bias_weight, 2))) +
theme_minimal()